What is a custom data type?
A custom data type is a data type used on a step property to render a specific field or set of fields. A data type might represent a simple text box field for input or a more complex popup UI for user selection.
Let's look at the configuration of the “Decision” step:
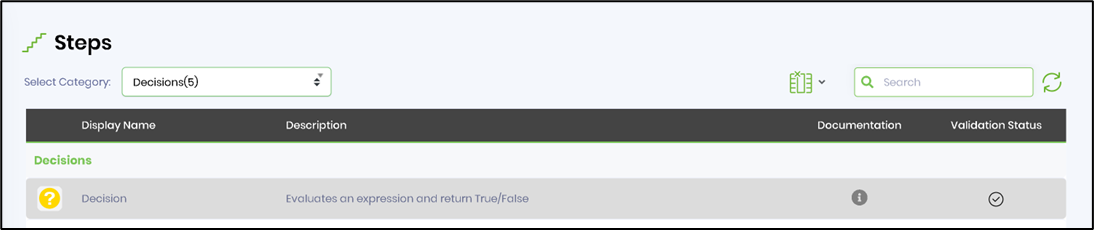
Configuration of the “Decision” step looks as follows:

About the above diagram, the “Decision” step has 1 property called “condition,” which is a “string” data type. When the step is rendered within the process designer, the “condition” property is rendered as a textbox.

Custom data types
Custom data types can be configured using the data type screen of the FlowWright Configuration Manager.
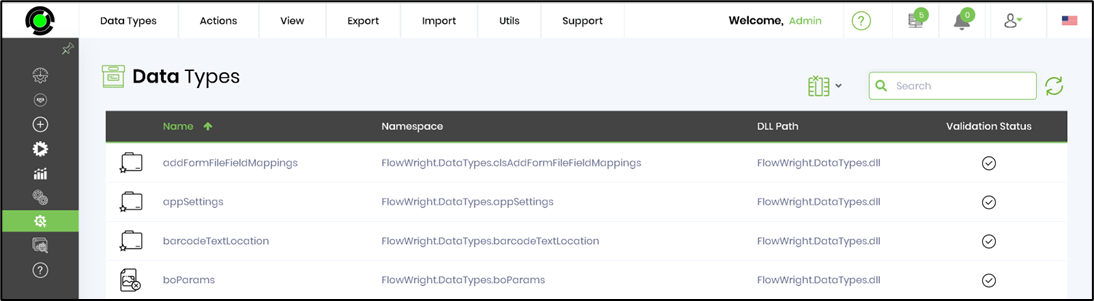
Select the “string” data type from the table and click on the View - Render menu option.
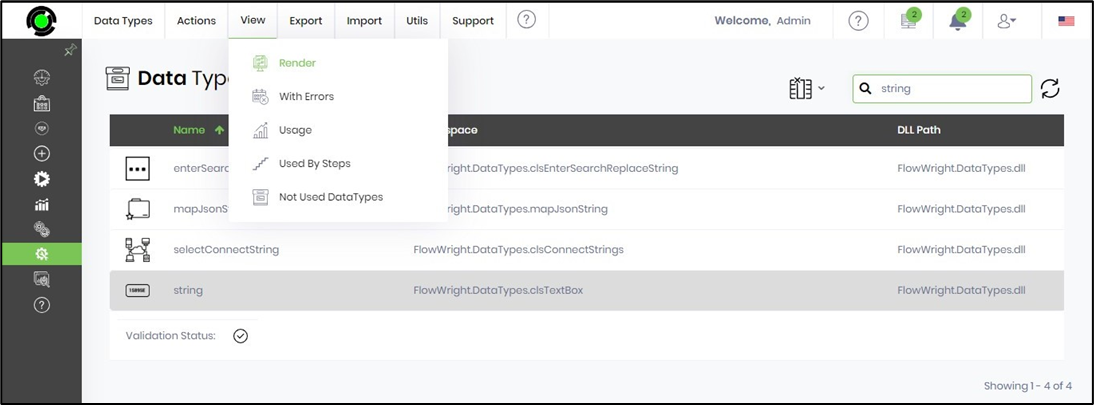
When the “string” data type is rendered, it looks as follows:

Writing a custom data type
Implementing the “IFWDataType” interface makes creating a custom data type easy. The following code shows the actual code for the “string” data type.
Here's an example video of how to build a custom data type:
Here's the code that was used in the custom data type:
using FlowWright.Engine; using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace FW10CustomItems { [DataTypeData("fwtextbox")] public class FWTextBox : IFWDataType { public string Render(FWDataTypeContext oDataTypeContext) { string sValue = ""; if (!string.IsNullOrWhiteSpace(oDataTypeContext.SelectedValue)) { sValue = oDataTypeContext.SelectedValue; } string sHTML = $"<input name='{oDataTypeContext.ControlID}' type='text' value='{sValue}' id='{oDataTypeContext.ControlID}'>"; return (sHTML); } } }